No trial. No credit card required. Just your GitHub account.
.NET Blog
Free. Cross-platform. Open source. A developer platform for building all your apps.
Featured posts
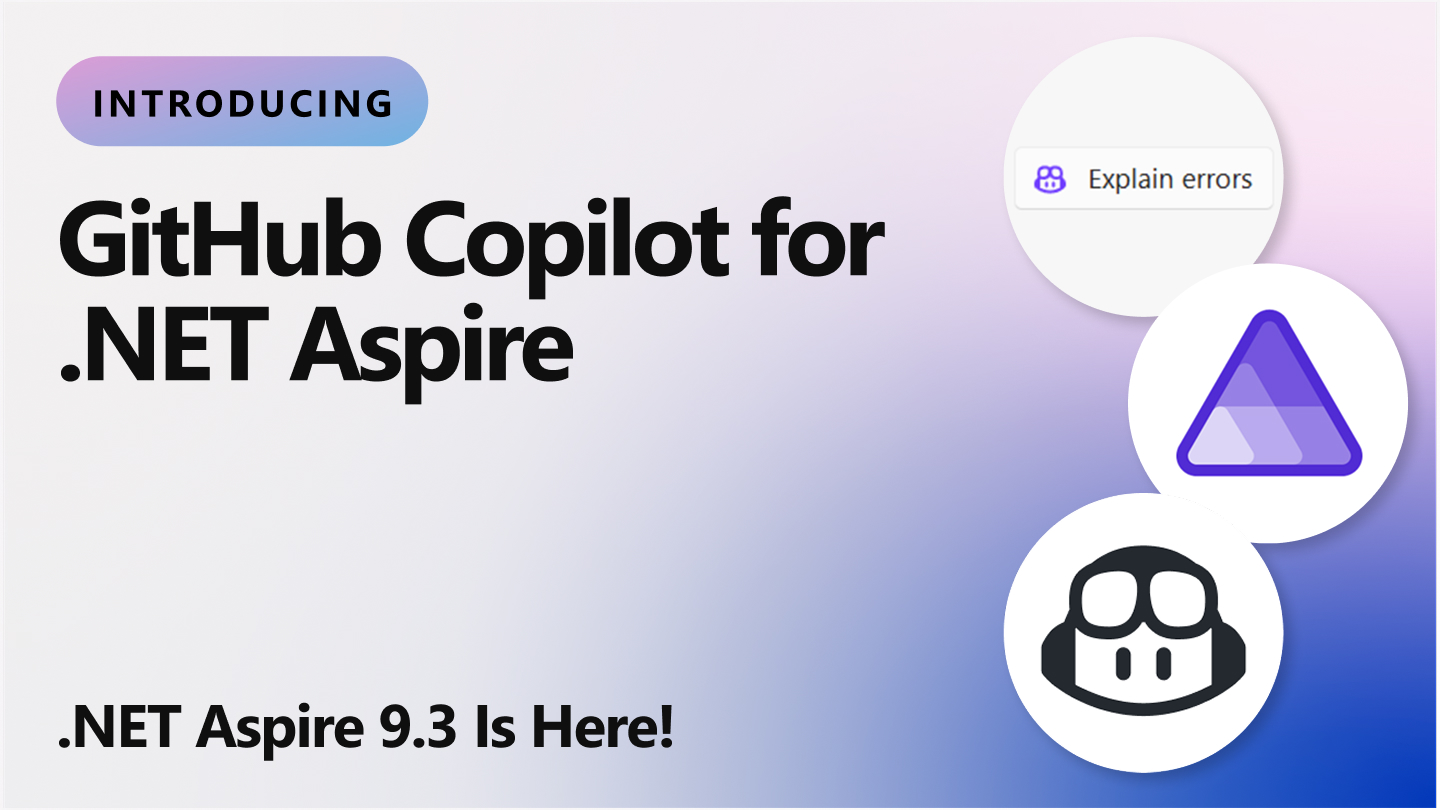
.NET Aspire 9.3 is here and enhanced with GitHub Copilot!
.NET Aspire 9.3 is the biggest release of .NET Aspire yet, with the introduction of GitHub Copilot directly into the .NET Aspire Dashboard, updates for integrat...
Latest posts
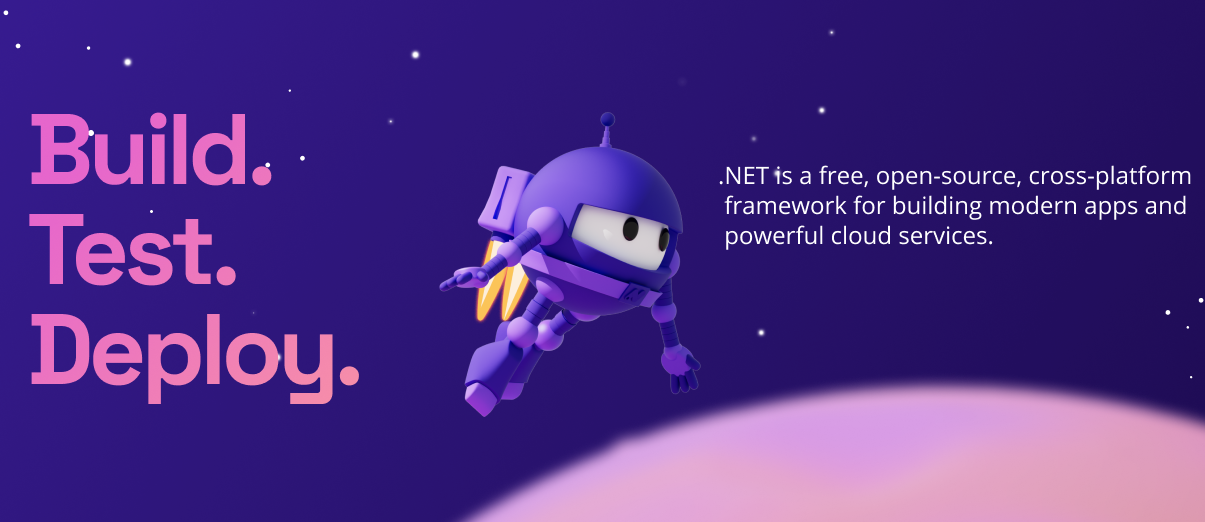
Introducing $select and $expand support in Web API OData

Last week Microsoft released the preview of Visual Studio 2013, and along with it came the ASP.NET and Web Tools for Visual Studio 2013 Preview. In this new release, we are expanding the OData support in Web API to include support for $select and $expand, two of the most popular OData query operators. In this blog post we’ll cover the following topics: What are $select and $expand The $select operator allows a client to pick a subset of the properties of an entity to be retrieved when querying a feed or a single entity. The $expand operator allows a client to retri...
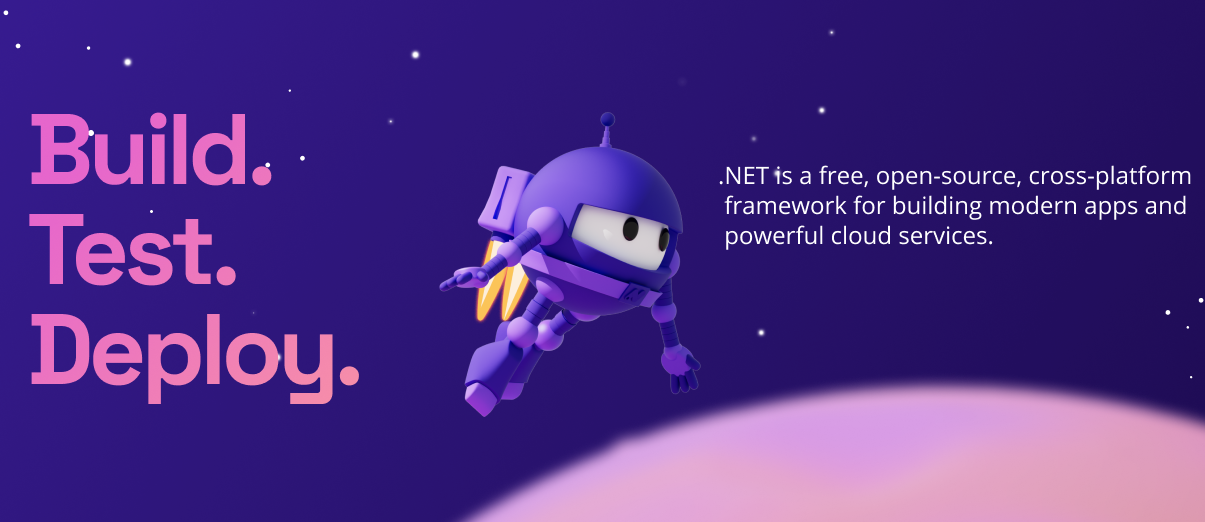
Understanding OWIN Forms authentication in MVC 5
Overview The new security feature design for MVC 5 is based on OWIN authentication middleware. The benefit for it is that security feature can be shared by other components that can be hosted on OWIN. Since the Katana team did a great effort to support the OWIN integrated pipeline in ASP.NET, it can also secure apps hosted on IIS, including ASP.NET MVC, Web API, Web Form. Forms authentication uses an application ticket that represents user's identity and keeps it inside user agent's cookie. When user first accesses a resource requiring authorization, it will redirect user to login page. After the user provides ...
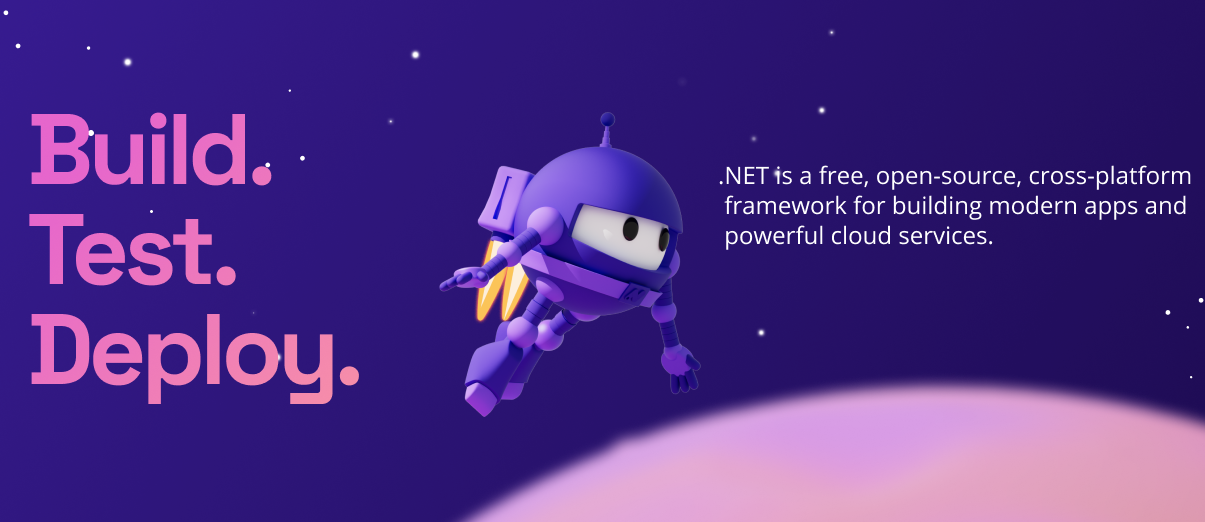
Manage CORS policy dynamically
Brief We introduced CORS support in ASP.NET Web API a few months ago. Out of the box it supports configuring CORS policy by attributes. It is a very intuitive and powerful way but lacks flexibility at runtime. Imaging your service allows a 3rd party to consume your service. You need the capability of updating the allowing origins list without compiling and deploying your service each time the list changes. In following this article, I show you two examples of dynamically managing your CORS policy. Prerequisites Set up the test environment CORS Service Create a WebAPI project. It co...
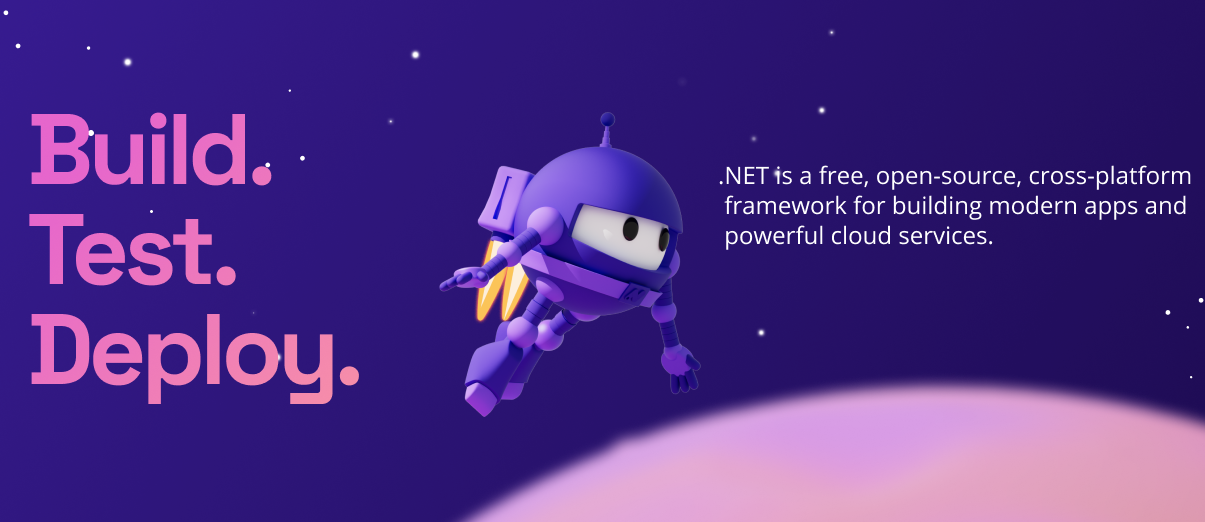
Fix SPA VB project creating issue for ASP.NET and Web Tools Preview Refresh for Visual Studio 2013 Preview
Issue Unfortunately after installing ASP.NET and Web Tools Preview Refresh for Visual Studio 2013 Preview, creating SPA VB template will fail. This post describes how to fix this issue. Option 1: Fix script I authored these two scripts (one for x86 and x64) to fix the issue. Download here Note: Option 2: Manually fix If for some reason you can’t run the scripts, you can fix the issue by altering template files.
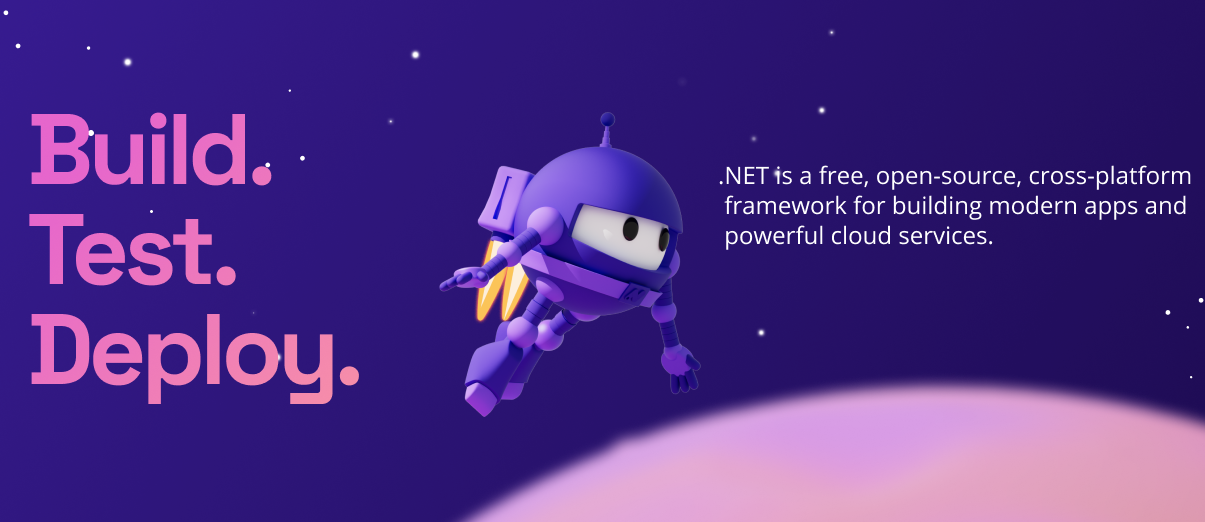
Make VS2012 MVC4 VSIX template to work for Visual Studio 2013 preview
Visual Studio 2012.2 supports customized MVC4 template, you can get a walkthrough here: http://blogs.msdn.com/b/yjhong/archive/2012/12/13/custom-mvc-4-template-walkthrough.aspx or http://www.asp.net/mvc/overview/getting-started/custom-mvc-templates. But the created template VSIX file cannot be uploaded to VS Gallery at the time. Starting 6/26/2013, you can upload the MVC4 template VSIX files to VS Gallery now.The VS2012.2 created VSIX may not install or work for Visual Studio 2013 preview, however. We need to fix a few thing in the following steps.1. Change source.extension.vsixmanifest file InstallationTarg...
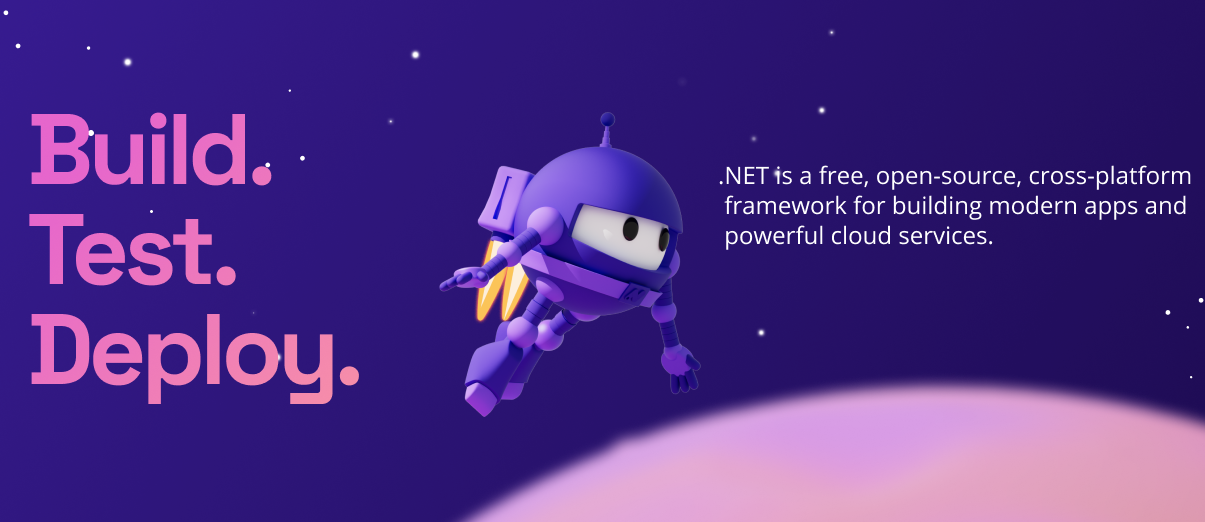
Browser Link feature in Visual Studio Preview 2013
Browser Link is just a channel between your Visual Studio IDE and any open browser. This will allow dynamic data exchange between your web application and Visual Studio. This feature is enabled by default in Visual Studio 2013 Preview. When Browser Link is enabled we register an HTTPModule with ASP.NET, which will then inject a <script> reference to every page request from the server. It is this JavaScript that does the magic of connecting the browser to Visual Studio. For Preview, we have a feature called the “Refresh Linked Browsers” that makes use of this channel between browsers and IDE. Because the b...
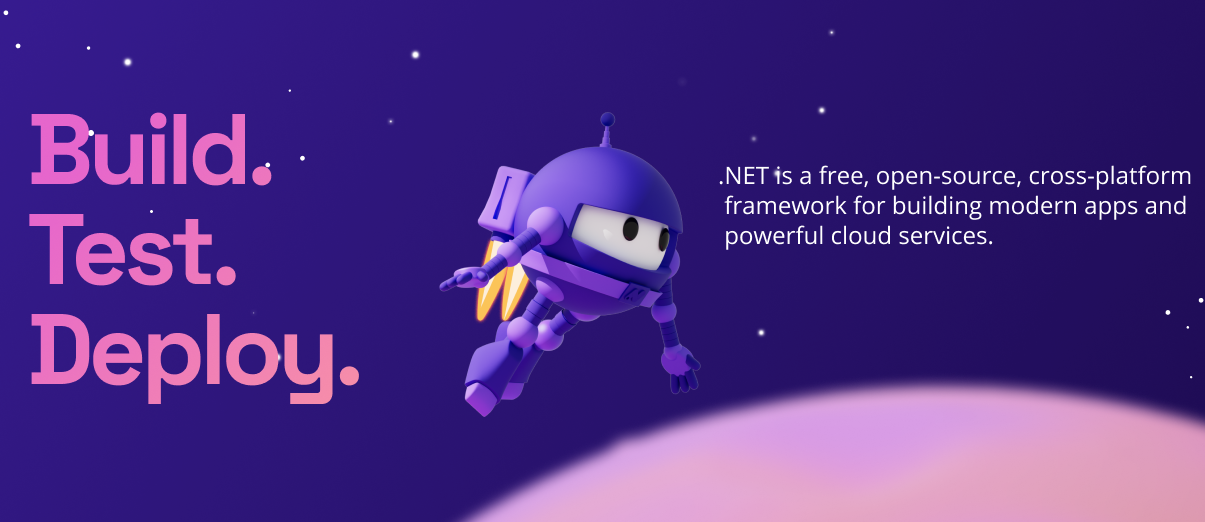
Introducing ASP.NET Identity – A membership system for ASP.NET applications
ASP.NET Identity is the new membership system for building ASP.NET web applications. ASP.NET Identity allows you to add login features to your application and makes it easy to customize data about the logged in user. [Update] Please visit ASP.NET Identity for getting the latest information about ASP.NET Identity project and learning more on how to get started and migrate from earlier membership systems. Features Following are some of the feature of the ASP.NET Identity system How do I get it? ASP.NET Identity is used by ASP.NET Web Fo...
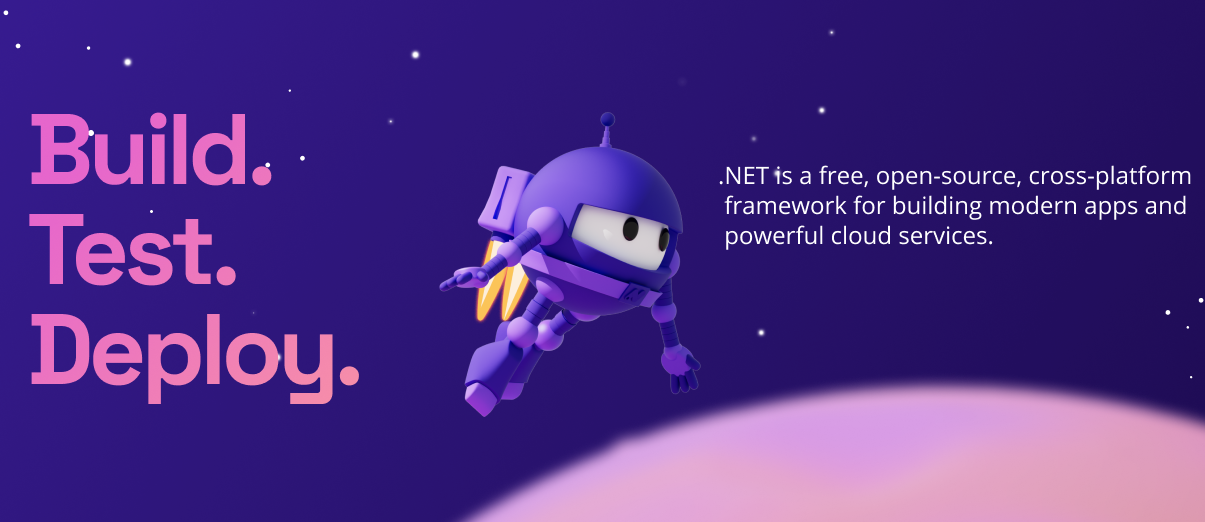
Get the latest .NET Bits

We maintain a set of pages that make it easier for you to find all the .NET Framework versions, SDKs, and targeting packs that you can take advantage of in your .NET apps, as we mentioned last year on the .NET blog. We recently redesigned the pages and added more SDKs for you to download. You may even find some SDKs to try that you didn’t know about. If you really just want the latest bits, use the following links: We've provided a separate page for each version of Visual Studio, since each version has a different set of capabilities: You can now quickly get to the .NET download tha...
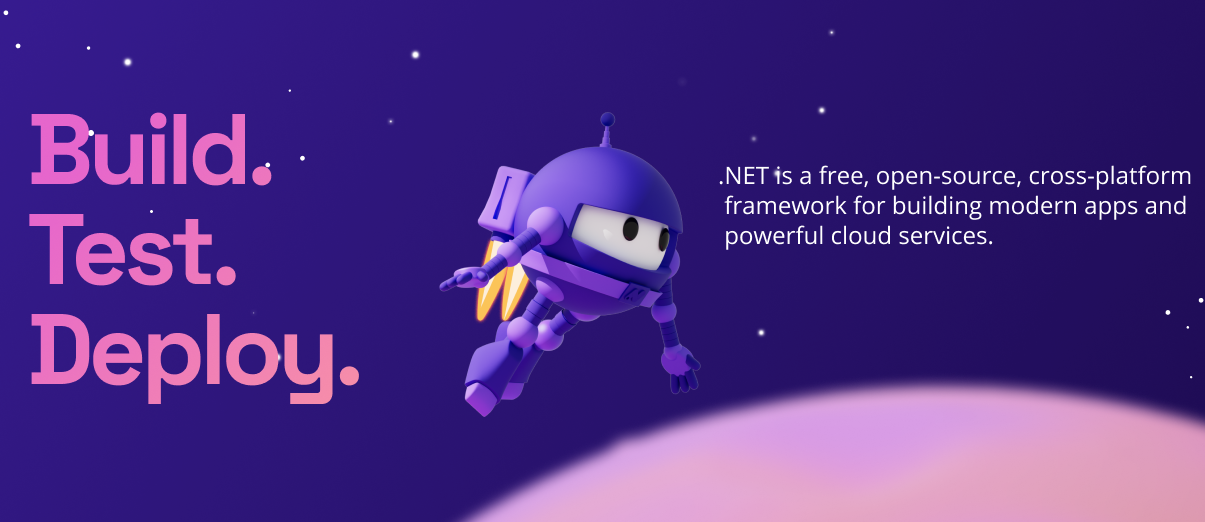
Adding External Logins to your ASP.NET application in Visual Studio 2013
The project templates showcase a way of logging in with external login providers, along with the normal way of logging in by creating a local account This post highlights how you can turn on support for logging through these services in the ASP.NET project templates that shipped with Visual Studio 2013 Preview. To learn more about how authentication via external providers works, please read the following article. http://www.asp.net/web-api/overview/security/external-authentication-services#USING Enable OAuth login using Facebook, Twitter Steps to get keys for Facebook Steps t...