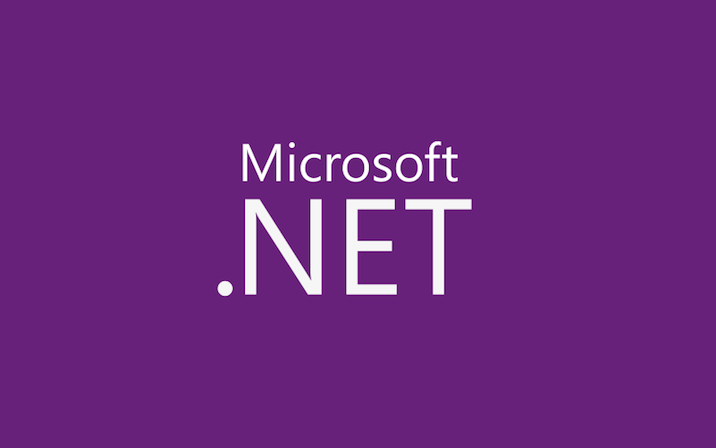
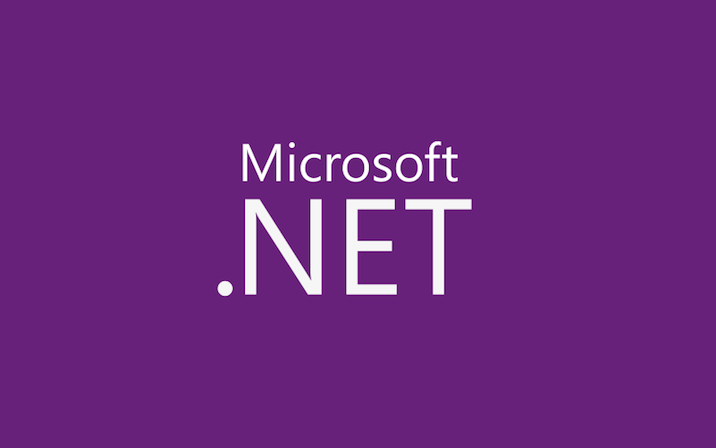
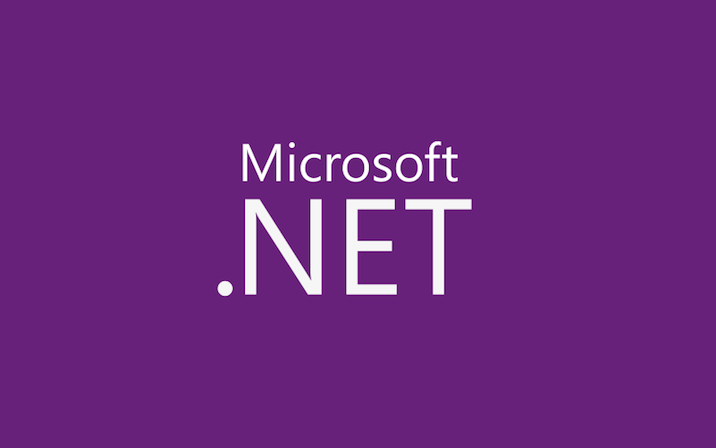
ParallelExtensionsExtras Tour – #13 – AsyncCall
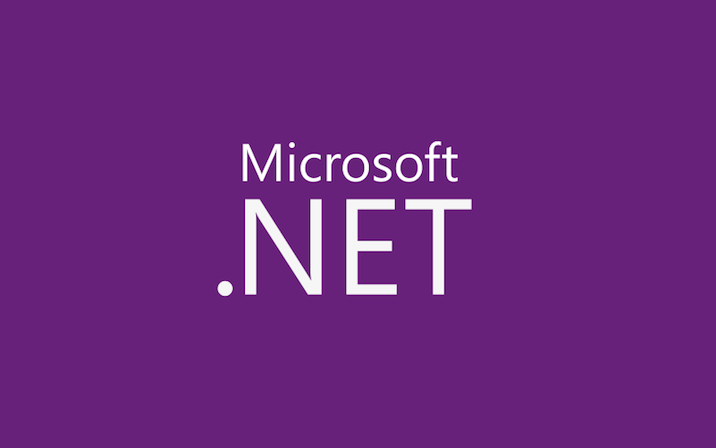
Performance of Concurrent Collections in .NET 4
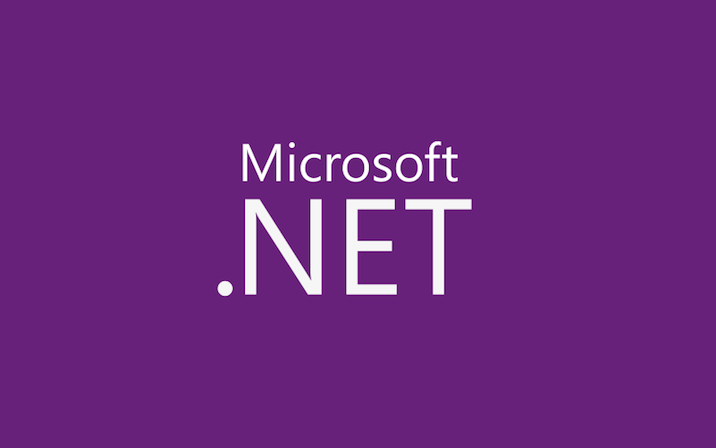
ParallelExtensionsExtras Tour – #12 – AsyncCache
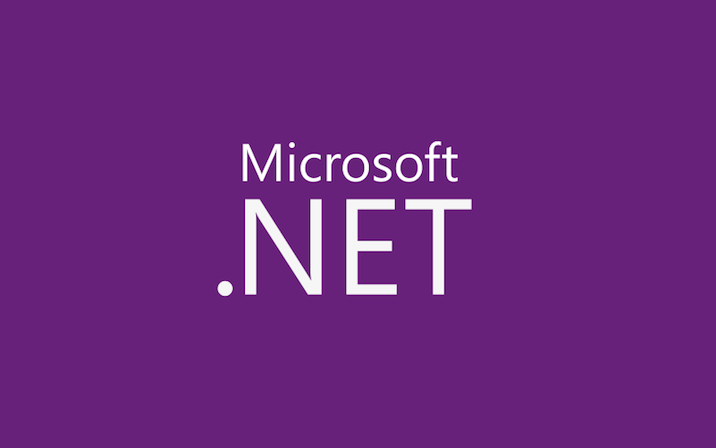
When To Use Parallel.ForEach and When to Use PLINQ
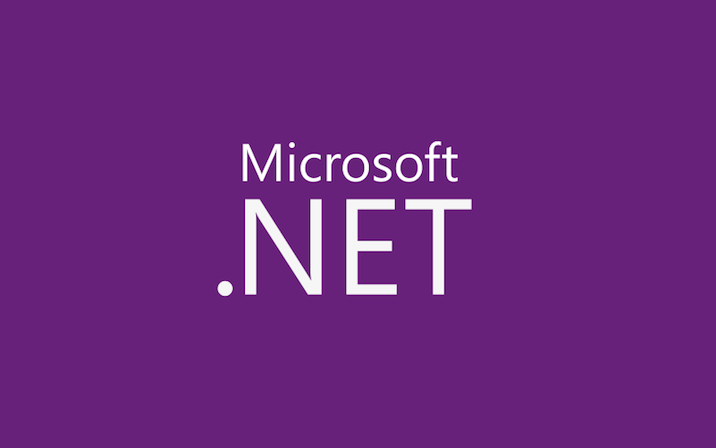
A Tour of Various TPL Options
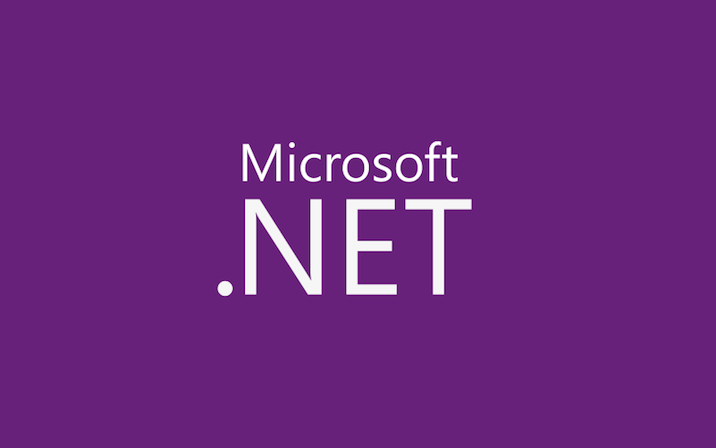
Parallel Computing Sessions at the Visual Studio 2010 Launch
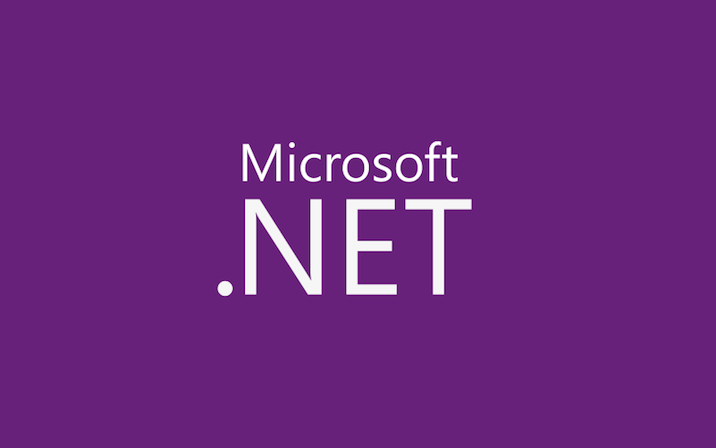
ParallelExtensionsExtras Tour – #11 – ParallelDynamicInvoke
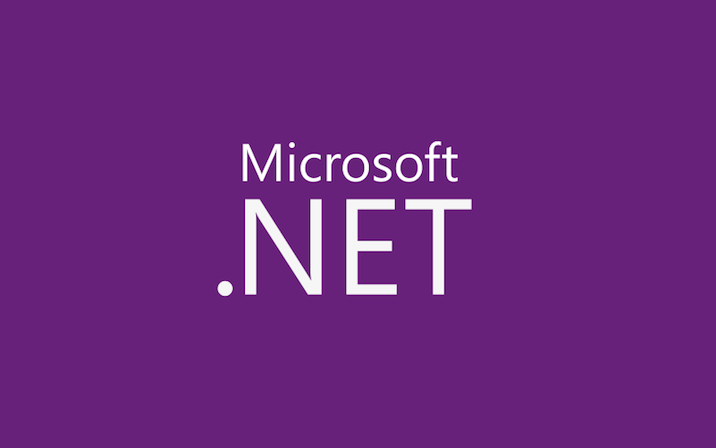
ParallelExtensionsExtras Tour – #10 – Pipeline
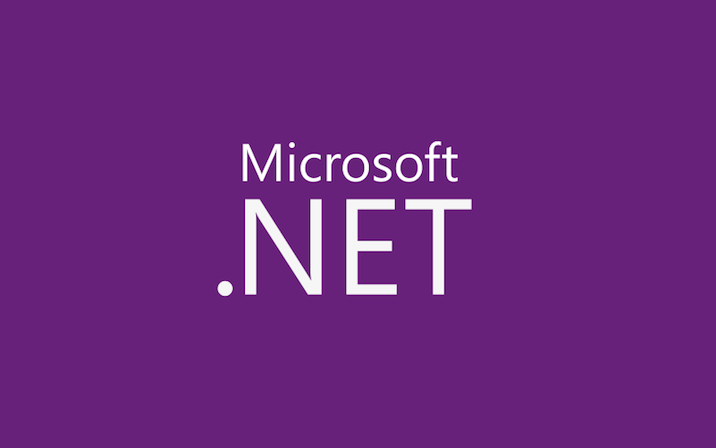