Beware the C++ implicit conversion
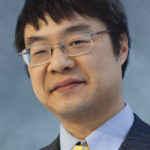
Today's topic was inspired by a question from a customer: I am working on a stack overflow bug. To reduce the size of the stack frame, I removed as many local variables as I could, but there's still a a lot of stack space that I can't account for. What else lives on the stack aside from local variables, parameters, saved registers, and the retur...