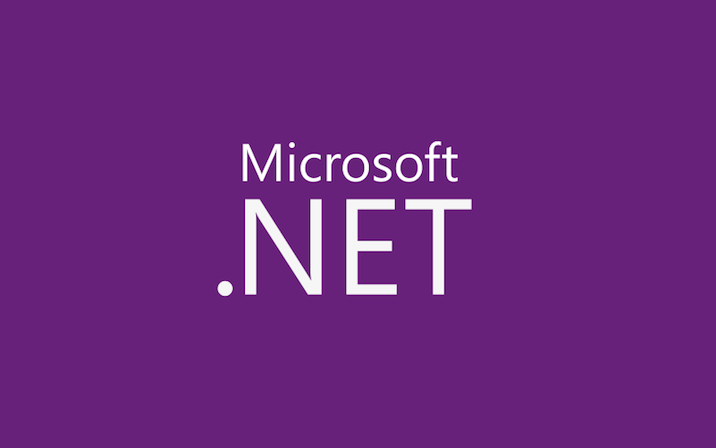
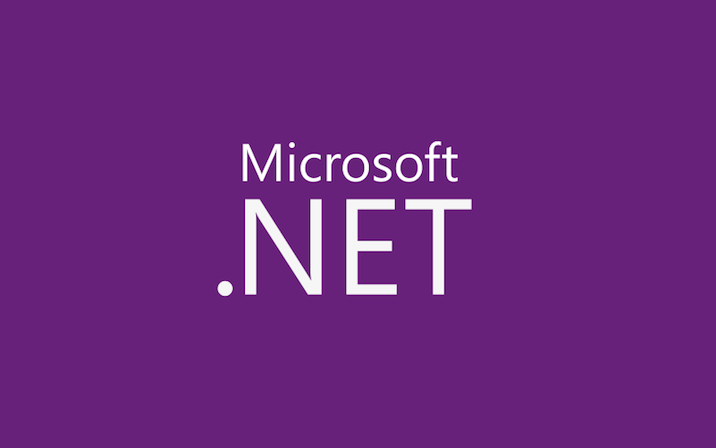
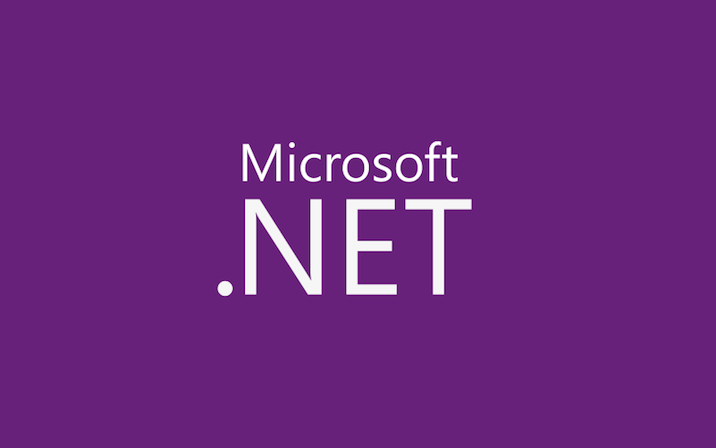
New “Parallel Computing” dev center on MSDN
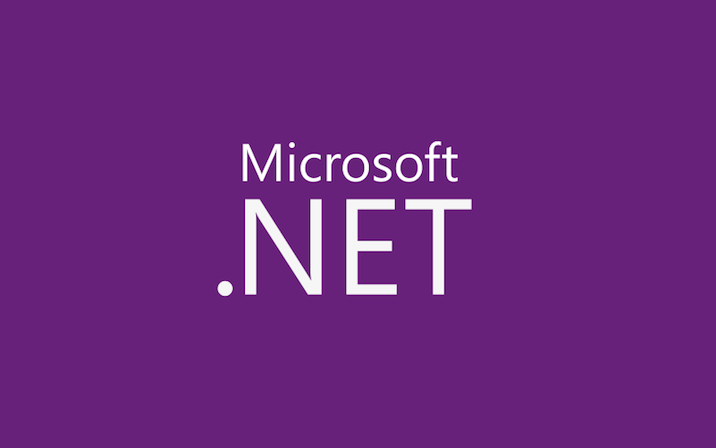
PLINQ changes since the MSDN Magazine article
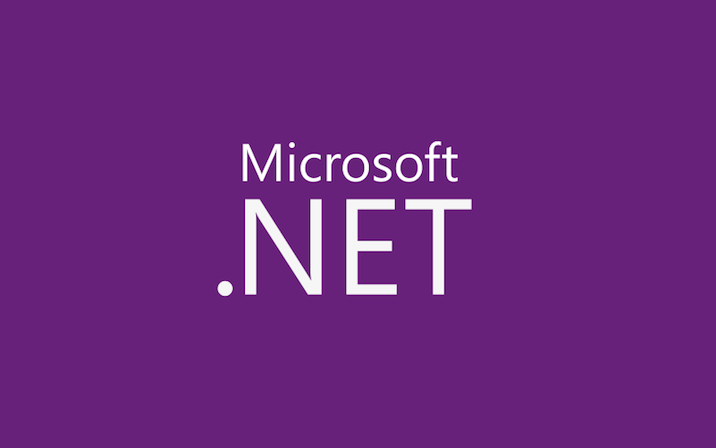
Task Parallel Library changes since the MSDN Magazine article
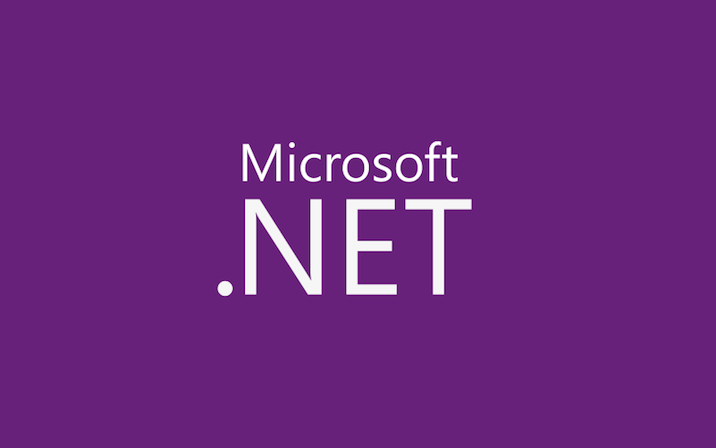
CTP Quality
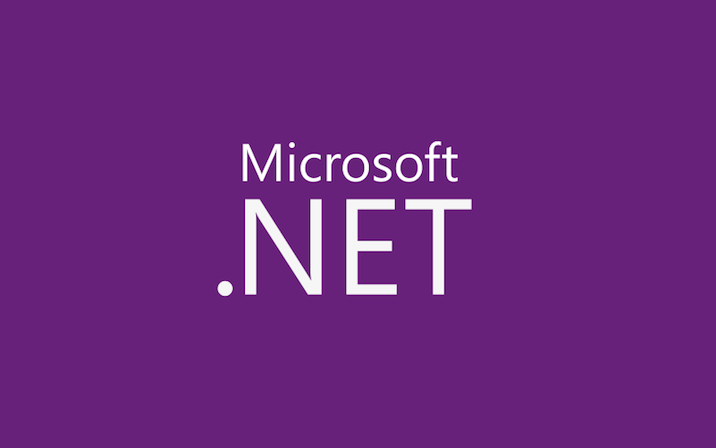
Welcome to the Parallel Extensions team blog!
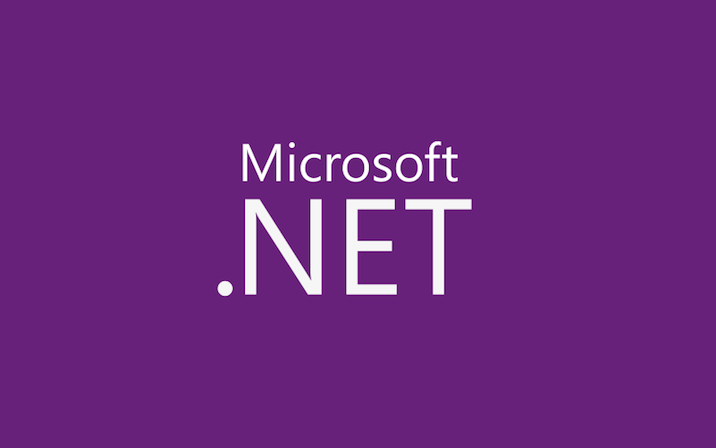
All about Async/Await, System.Threading.Tasks, System.Collections.Concurrent, System.Linq, and more…