Post by this author
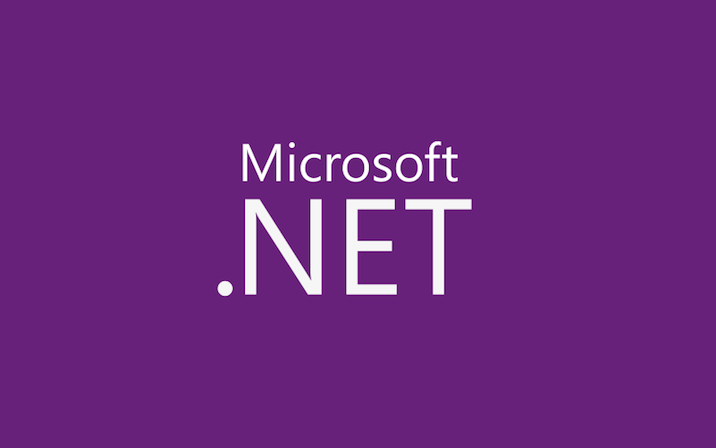
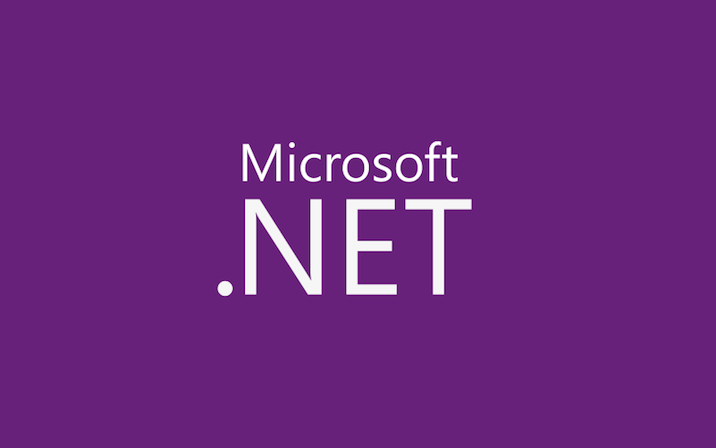
Await, SynchronizationContext, and Console Apps
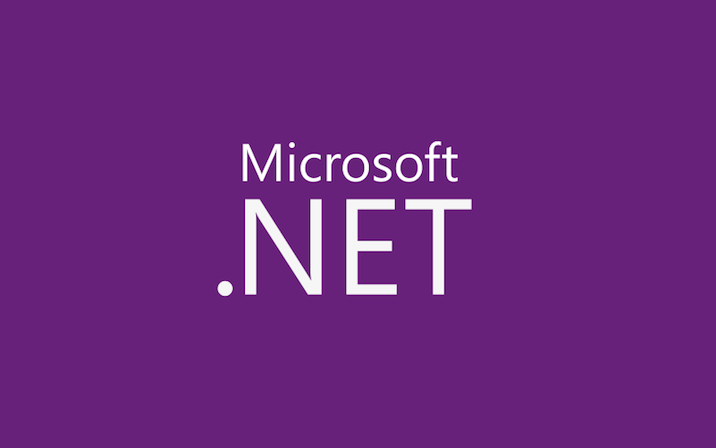
FAQ on Task.Start
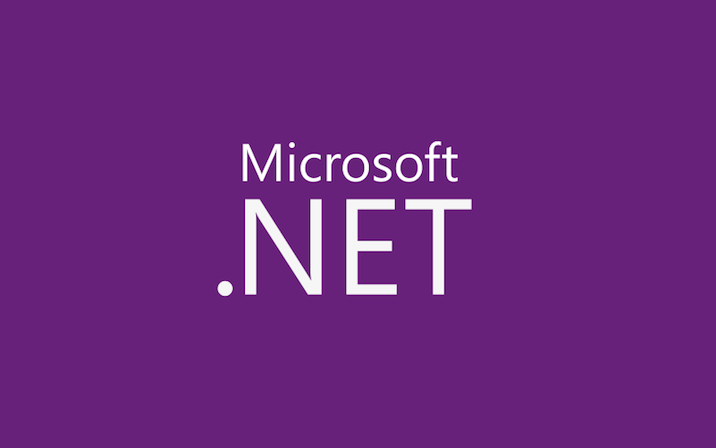
Awaiting Socket Operations
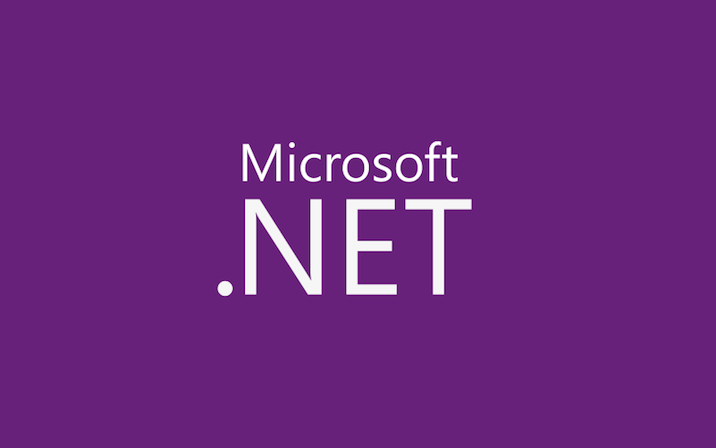
Coalescing CancellationTokens from Timeouts
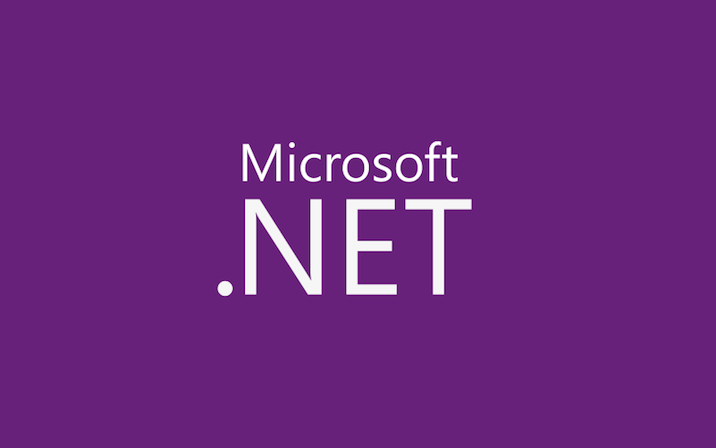
Updated Async CTP
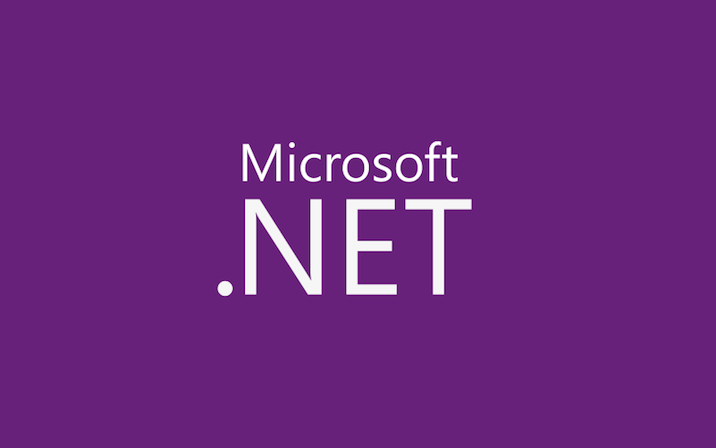
When at last you await
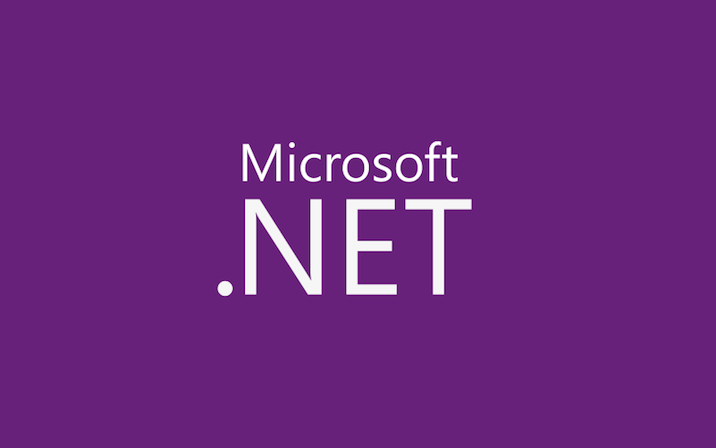
Task.Run vs Task.Factory.StartNew
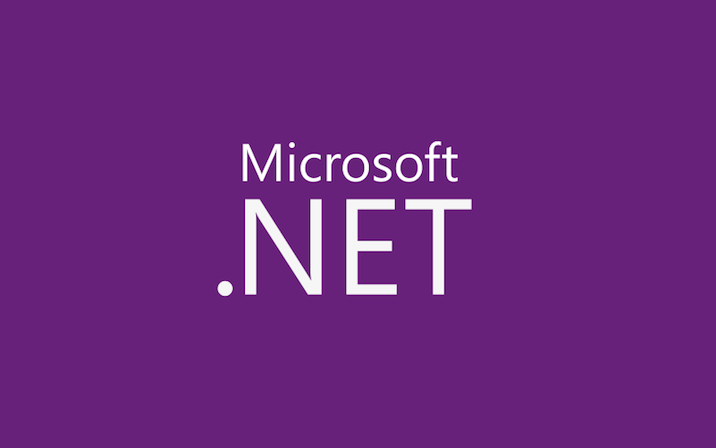
New articles on async/await in MSDN Magazine
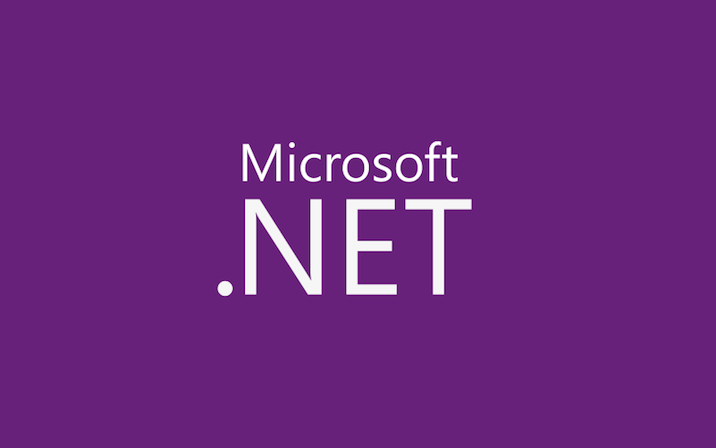