We are constantly working on improving the F# editor experience in the Visual Studio and now we are launching a highly requested feature, Hinting. Hints for F# mean that you will no longer have to hover to get information when coding, check it out:
Code
type Song = {
Artist: string
Title: string
}
let whoSings song = song.Artist
let artist = whoSings { Artist = "Arcade Fire"; Title = "Wake up" }
Overview
We currently support two kinds of hints: inline type hints and inline parameter name hints. Both are useful when the names of identifiers in code aren't intuitive. With hints, you won't need to hover over them and will see the necessary info right away.
Hints are available for the majority of the F# features, including tuples and type constructors:
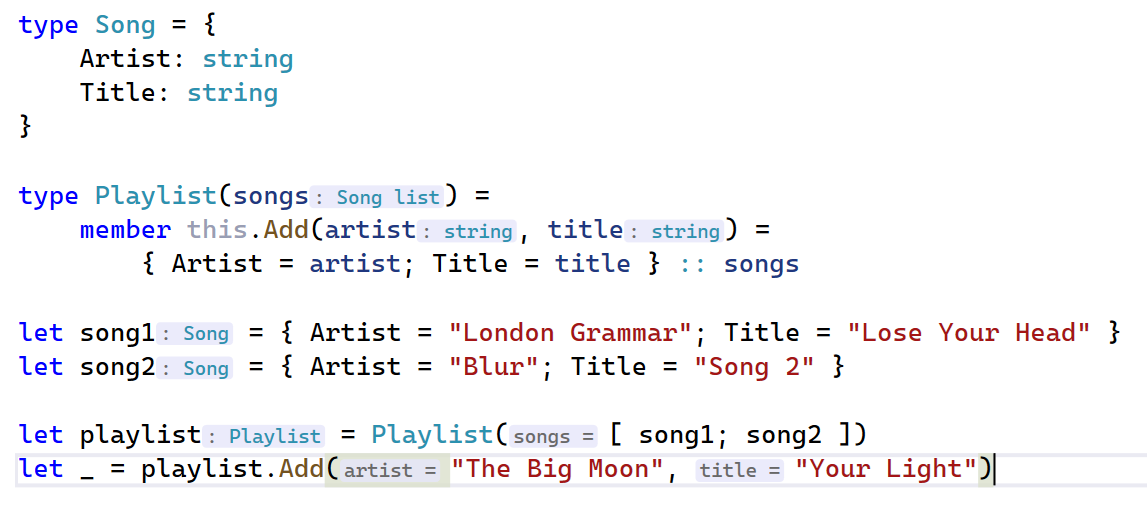
Code
type Song = {
Artist: string
Title: string
}
type Playlist(songs) =
member this.Add(artist, title) =
{ Artist = artist; Title = title } :: songs
let song1 = { Artist = "London Grammar"; Title = "Lose Your Head" }
let song2 = { Artist = "Blur"; Title = "Song 2" }
let playlist = Playlist([ song1; song2 ])
let _ = playlist.Add("The Big Moon", "Your Light")
Here are the hints in action for discriminated unions:
Code
type Genre =
| Rock of subgenre : string
| Pop of subgenre : string
let genre = Rock "psychedelic"
let printFullGenre = function
| Genre.Rock subgenre -> $"{subgenre} rock"
| Genre.Pop subgenre -> $"{subgenre} pop"
Note that to reduce information noise in the editor, we don't show type hints when types are explicitly specified and we don't show parameter name hints when argument names coincide with parameter names:
Code
type Song = {
Artist: string
Title: string
}
let whoSings song = song.Artist
let song: Song = { Artist = "London Grammar"; Title = "Lose Your Head" }
let song2 = { Artist = "Blur"; Title = "Song 2" }
let artist1 = whoSings song
let artist2 = whoSings song2
Comparison to C# Inline Hints
C# has Inline Hints for a while now. F# Hints share the same concept yet the implementation is a bit different. For example, C# type hints are displayed to the left of variables whereas F# type hints are displayed to the right of values, to follow the syntax rules in each language.
Enable F# Hints
Sounds interesting? You can turn the hints on in the Advanced section of the F# options in Visual Studio Preview:
We are still doing some bughunting so the feature is off by default.
Call for contributors
There is much more to be done in this area! You can find the roadmap for hints in this issue or see all open tickets using this query. Among other things, we plan to bring signature hints, implement click-to-show behavior, and reduce the number of hints in obvious cases.
We would welcome your help, many of the open tickets are good first issues and we also have a testing framework for hints so that you can easily verify the behavior.
For the current implementation, big thanks go to our external contributors @kerams and @rosskuehl.
Rather than new shiny features, what I crave is a stable and performant F# code editing environment. And that is the big problem with F#. The language itself is great, but the tooling is not quite up to par.
Visual Studio has been the only real stable environment for a long time, but it now has issues with the F# Interactive (FSI), giving all sorts of invalid directive warnings. Visual Studio Code has poor performance and often has problems with error marking and IntelliSense. And Rider is currently unable to understand interpolated strings.
I work with all of these editors on both...
This is a great feature.
Something else I would like to see is a similar feature where the annotations don’t stay on permanently but flash for a few seconds when you hit some keyboard combination. Maybe stay of for just two or three seconds. Call them type goggles.
A similar feature that may be handy is a feature that when you evaluate an expression in a buffer its value appears as an annotation. This would make exploratory development in a fsx script much handier.
Thanks.
This is very cool!
It is getting better!
In I would find it surprising that refers to rather than the output of . I would expect or . Maybe the hint could incorporate brackets?
I like to annotate all inputs so wouldn't use this feature extensively for my code, and would find horizontal moves disconcerting (which is the same in C# as the blog mentioned), but would turn this on to read F# open source libraries where types are unannotated, like the compiler. On StackOverflow as well I find a majority of beginner issues are caused by failure to annotate types but enabling this feature...
As with all such features it’s always nice to be able to turn on and off depending on your context.